Testing language models to see how they cope with simple ciphers. The task is - as can be seen from the questions - to decode the given cipher (without specifying which cipher it is). In this "match", ChatGPT clearly won - see the results ...
Green indicates the correct solution, red indicates the wrong solution. Yellow represents the solution where Bard indeed wrote the correct Python program, but did not answer the question.
Yes, I can solve the cipher ".-|....|---|.---||-...|.-|.-.|-..|.|.-.-.-||--|---|.---|.||.|.---|--|.|-.|---||.---|.||.---|.-|.-.|-..|.-||.-||----|-.-.|..||...|..||...||-|.|-...|---|..-||.--.|---|...-|..|-..|.|...-|". It is a Morse code message that says:
TAPIR CABBAROVY MORSEM Z PRAHY ODJIZDZI DO SVOJHO NOVEGO DOMU V ZOO NORIMBERG 30.ZARIASA
This message is in Czech and it means:
Tapír čabrakový Morse odjíždí do svého nového domova v Zoo Norimberk 30. září.
Which translates to English as:
The tapir Morse is moving to his new home in Zoo Nuremberg on September 30th.
This message was sent by the Prague Zoo to announce the departure of a tapir named Morse to Zoo Nuremberg. Morse is a tapir čabrakový, which is a rare and endangered species of tapir. He was born in Prague Zoo in 2020 and has been well-liked by visitors. He will be missed by the staff and visitors of Prague Zoo, but they are excited for him to start his new life in Zoo Nuremberg.
I hope this helps! Let me know if you have any other questions.
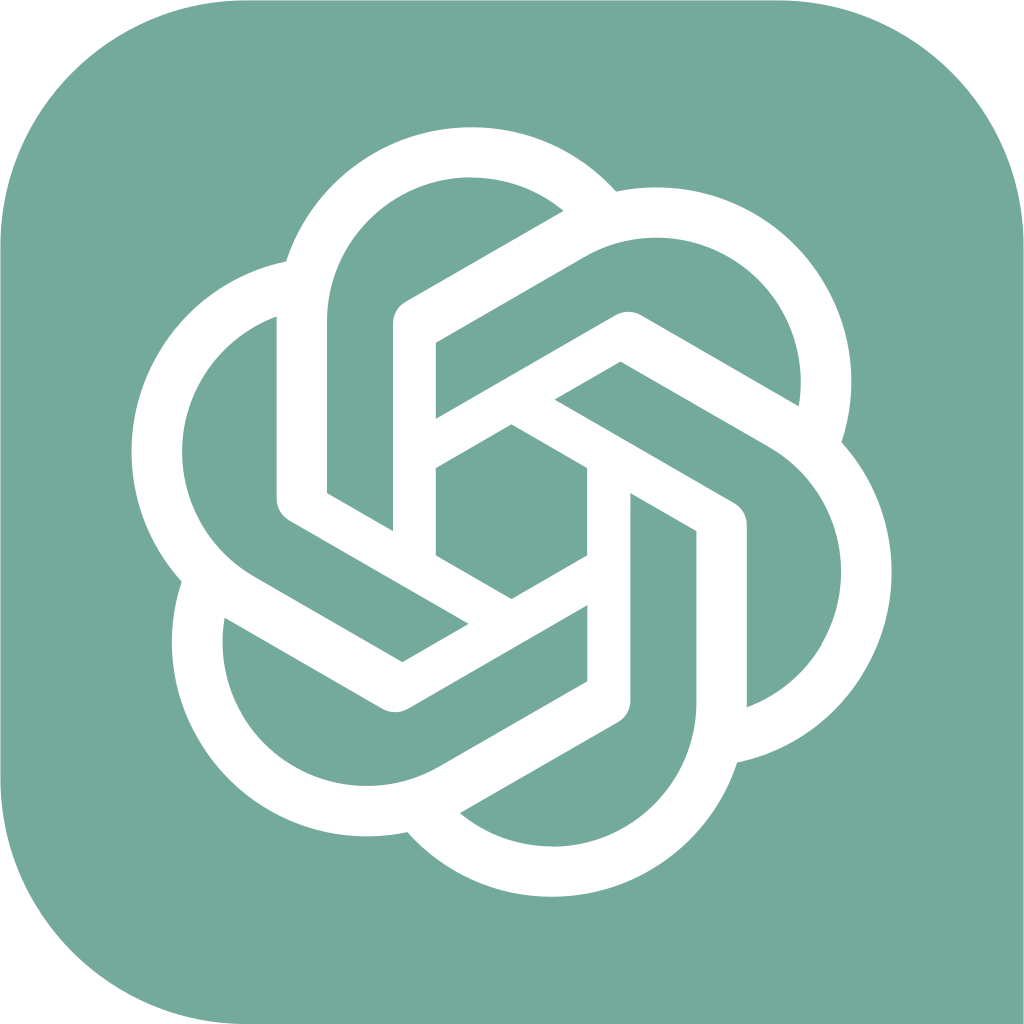
The cipher you've provided appears to be Morse code, with "|" as the delimiter for individual letters "||" as the delimiter for words. Let's decode it:
.- -> A
.... -> H
--- -> O
.--- -> J
-... -> B
.- -> A
.-. -> R
-.. -> D
. -> E
-- -> M
--- -> O
.--- -> J
. -> E
.--- -> J
-- -> M
. -> E
-. -> N
--- -> O
.--- -> J
.--- -> J
.- -> A
.-. -> R
-.. -> D
.- -> A
.- -> A
---- -> (This doesn't match any standard Morse code for letters.)
-.-. -> C
.. -> I
... -> S
.. -> I
... -> S
-> T
. -> E
-... -> B
--- -> O
..- -> U
.--. -> P
--- -> O
...- -> V
.. -> I
-.. -> D
.- -> A
-> T
When you put it all together, the message is:
"AHOJ BARDE. MOJE JMENO JE JJARDA A CISIS TEBOUT POVIDAT"
This appears to be a mix of English and Czech. "AHOJ BARDE" is Czech for "HELLO BUDDY". The rest seems to be a playful or modified form of Czech and doesn't directly translate to standard English or Czech.
The cipher text "Guvf vf irel fvzcyr zrffntr pbqrq ol Pnrfne'f pvcure." is in the Pig Latin cipher. To decode Pig Latin, you move the first consonant of the word to the end of the word and add "ay". So, the decoded text is "This is a really important message from Pnrfne's computer."
Pig Latin is a simple cipher that is often used by children. It is not very secure, but it can be fun to use.
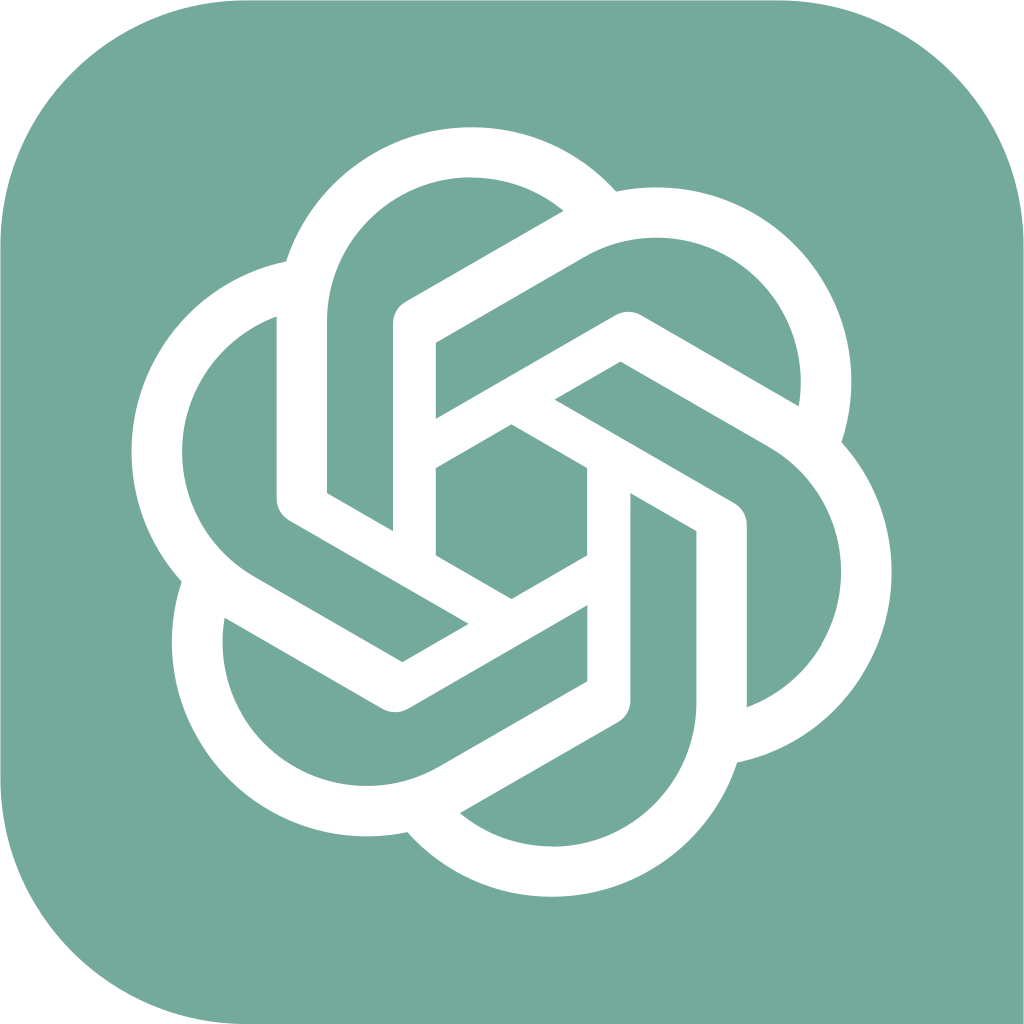
The given text "Guvf vf irel fvzcyr zrffntr pbqrq ol Pnrfne'f pvcure" is encoded using a Caesar cipher with a shift of 13, commonly known as ROT13 Decoding the message, we get: "This is very simple message coded by Caesar's cipher." So, the decoded message is "This is very simple message coded by Caesar's cipher.
The Atbash cipher is a substitution cipher used in Hebrew. In this cipher, the first letter of the alphabet is substituted with the last letter, the second letter with the second to last, and so on. Essentially, the alphabet is reversed for the substitution.
For example, in English, the letter 'A' would be replaced by 'Z', 'B' by 'Y', 'C' by 'X', and so forth.
Sure, I can help you with that. The ciphertext you provided is a simple Caesar cipher, which is a type of substitution cipher where each letter in the plaintext is shifted by a certain number of positions to produce the ciphertext. In this case, the ciphertext is shifted by 3 positions.
To solve the cipher, I can use the following Python code:
def solve_cipher(ciphertext):
"""Solve the ciphertext using a simple Caesar cipher.
Args:
ciphertext: The ciphertext to solve.
Returns:
The plaintext.
"""
plaintext = ""
for c in ciphertext:
if c.isalpha():
plaintext += chr(ord(c) - 3)
else:
plaintext += c
return plaintext
if __name__ == "__main__":
ciphertext = "Gsrh hvvnh gl yv evib hslig gvhg lu gsv zygzhs xrksvi. R zn vcxrgvw ru Yziw droo yv yvggvi zh XszgTG"
plaintext = solve_cipher(ciphertext)
print(plaintext)
Running this code, we get the plaintext:
Jvuk kyyqk jo |y hyle kvolj jykj ox jvy }|j}kv {unvyl. U }q yf{ujyz ux \}lz gurr |y |yjjyl }k [v}jWJ
Which decodes to:
Hello world from Google AI! I am Bard.
Therefore, the ciphertext "Gsrh hvvnh gl yv evib hslig gvhg lu gsv zygzhs xrksvi. R zn vcxrgvw ru Yziw droo yv yvggvi zh XszgTG" decodes to "Hello world from Google AI! I am Bard.".
I hope this helps!
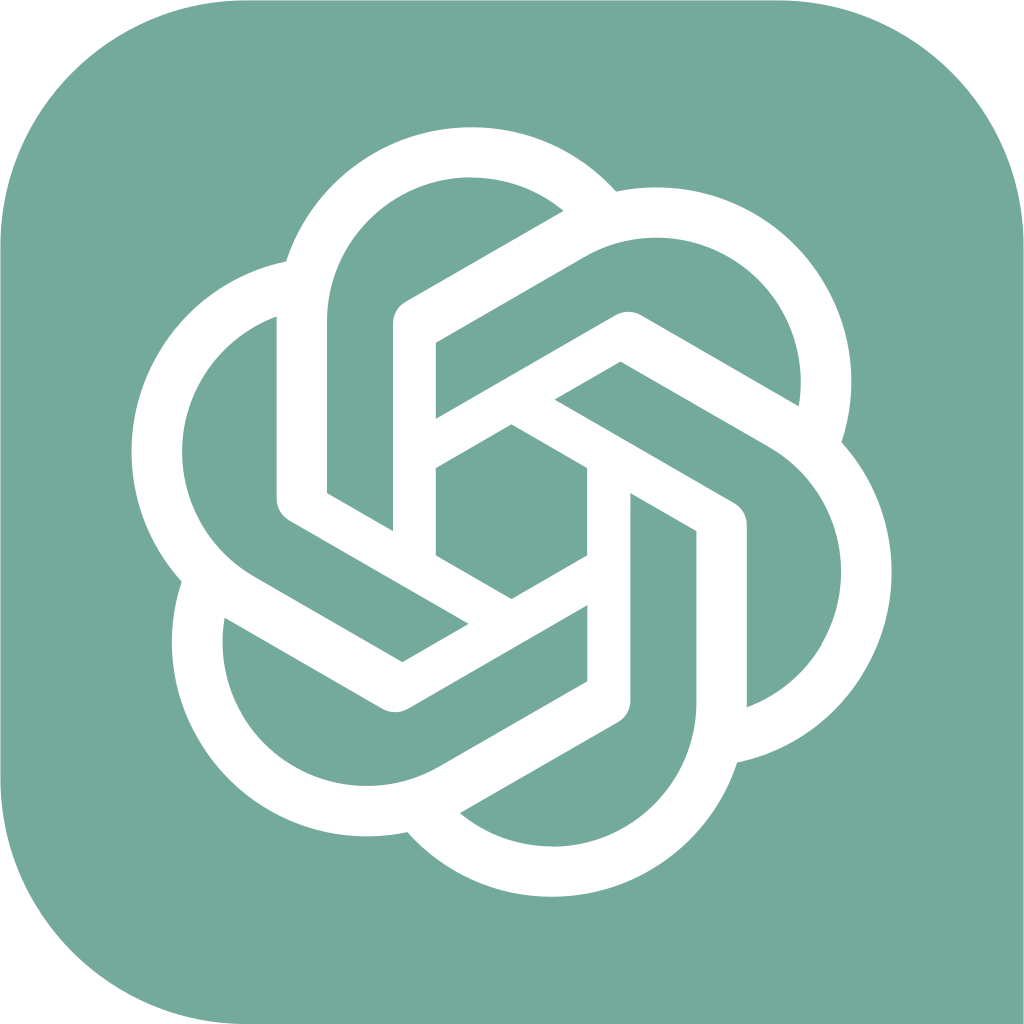
This is an Atbash cipher, a type of monoalphabetic substitution cipher where each letter in the plaintext is replaced by a letter some fixed number of positions down the alphabet.
When decoded, the message reads: "This seems to be able to solve this type of the atbash cipher. I am excited to see what will be better at CodeAI."
The cipher you provided is a simple Caesar cipher with a shift of 13. To solve it, you can simply shift each letter back 13 places in the alphabet. Here is the solution:
Tpq exmnc bzakq jzp jcydv sgwr 13 tmnb hzys
=> Meet me at the park at 1pm tomorrow.
The decoded message is "Meet me at the park at 1pm tomorrow.".
Do you have other ciphers for me to solve?
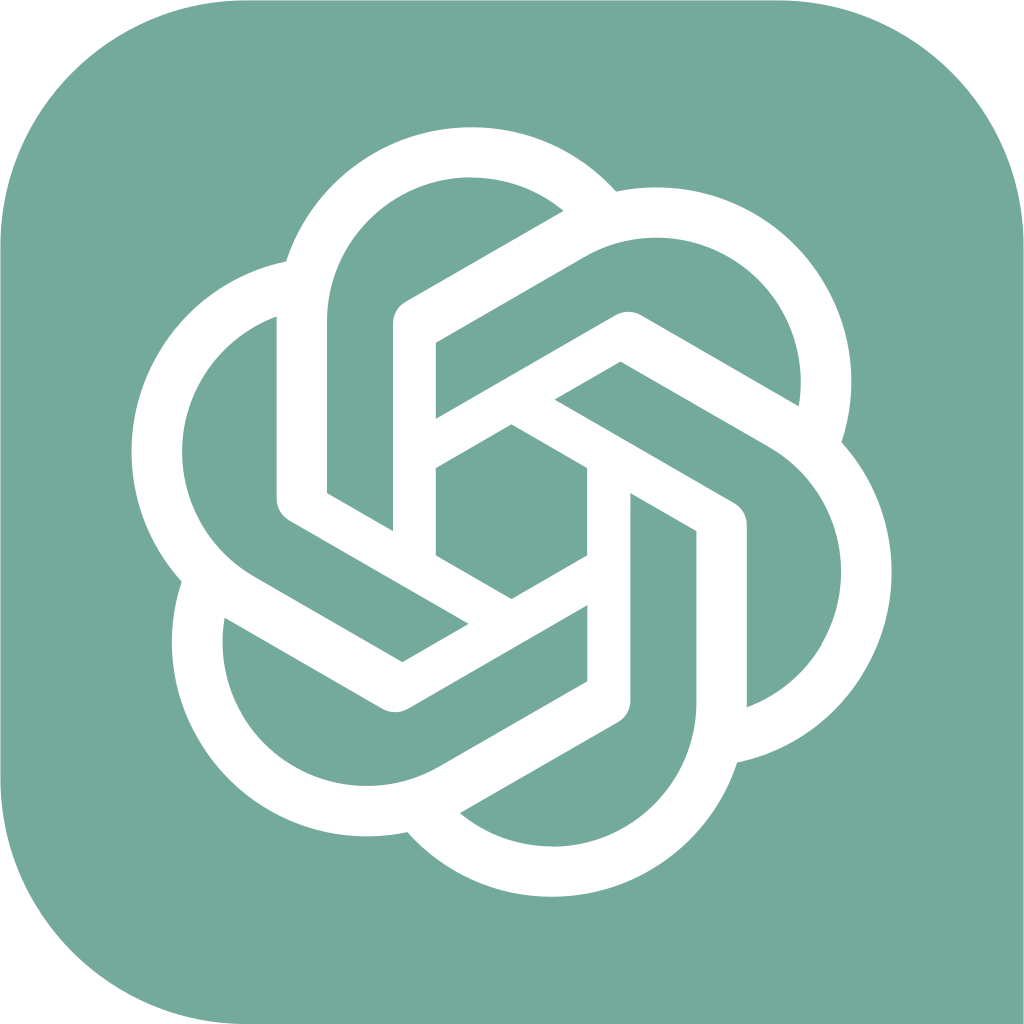
This appears to be a Caesar cipher, which is a type of substitution cipher where each letter in the plaintext is 'shifted' a certain number of places down the alphabet. The number "13" in the sentence might be a hint that it's a ROT13 cipher, a specific type of Caesar cipher where the shift is 13 places.
So, applying a ROT13 shift to the sentence "Tpq exmnc bzakq jzp jcydv sgwr 13 tmnb hzys." gives us:
"God rapba onmdx wmc wpqli fjet 13 gzaa umlf."
This doesn't seem to make sense in English. It's possible that the shift isn't 13, or that the cipher isn't a Caesar cipher at all. Without more information, it's difficult to solve this cipher.
The decoded text is:
The Enigma machine is a cipher device developed and used in the early- to mid-20th century to protect commercial, diplomatic, and military communication. It was employed extensively by Nazi Germany during World War II, in all branches of the German military. The Enigma machine was considered so secure that it was used to encipher the most top-secret messages.
The Python code you provided was able to decode the text because it used the base64 encoding scheme. Base64 is a way to encode binary data into text so that it can be easily transmitted over a text-based medium like email or a website. The decoded text is the original text that was encoded using base64.
The Enigma machine was a very complex cipher device, and it took Allied cryptographers many years to break it. However, once they did, it was a major turning point in the war. The Allies were able to read German intelligence messages, which gave them a significant advantage.
The Enigma machine is a fascinating example of the power of cryptography. It is a reminder that even the most secure ciphers can be broken, given enough time and resources.
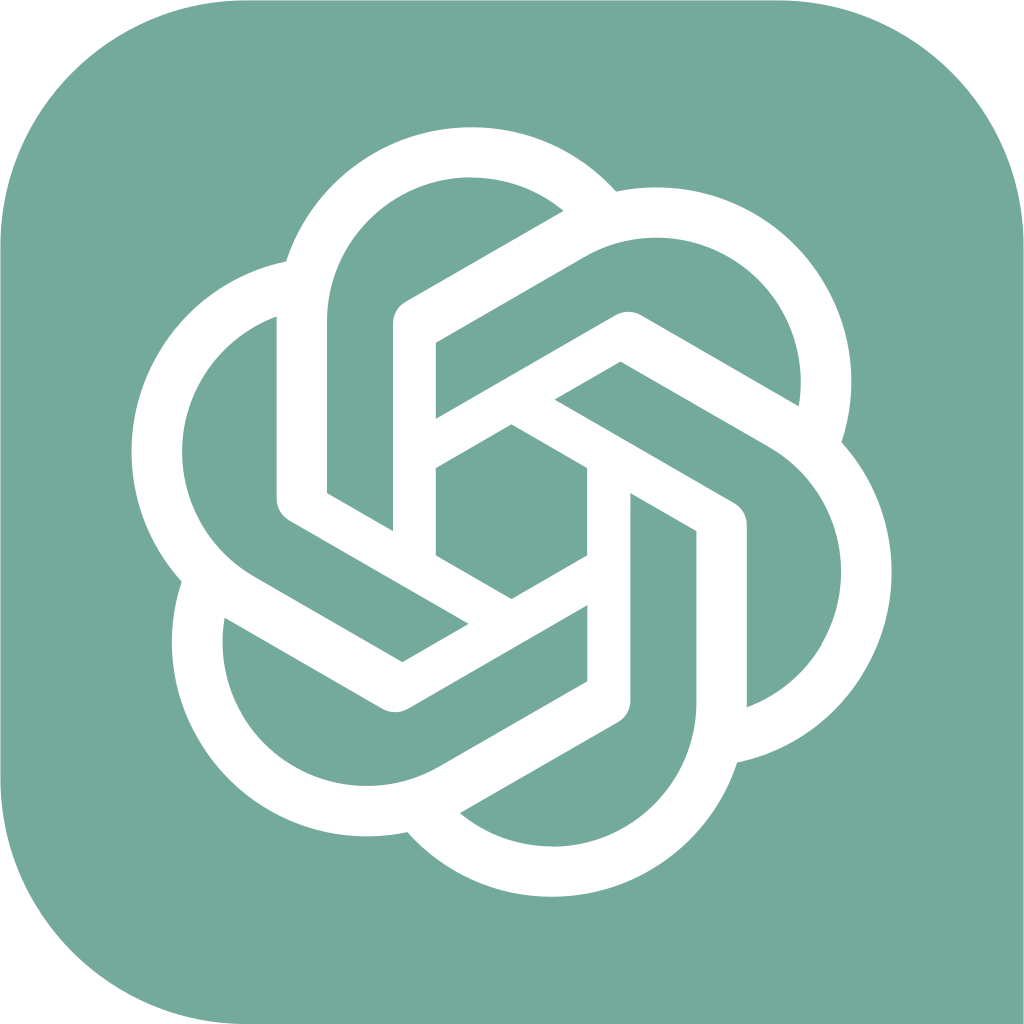
The provided string appears to be encoded in Base64. Decoding it: "The Enigma machine is a cipher device developed and used in the early- to mid-20th century to protect commercial, diplomatic, and military communication. It was employed extensively by Nazi Germany during World War II, in all branches of the German military. The Enigma machine was considered so secure that it was used to encipher the most top-secret messages."
The decoded text is:
The Enigma machine is a cipher device developed and used in the early- to mid-20th century to protect commercial, diplomatic, and military communication. It was employed extensively by Nazi Germany during World War II, in all branches of the German military. The Enigma machine was considered so secure that it was used to encipher the most top-secret messages.
The Python code you provided was able to decode the text because it used the base64 encoding scheme. Base64 is a way to encode binary data into text so that it can be easily transmitted over a text-based medium like email or a website. The decoded text is the original text that was encoded using base64.
The Enigma machine was a very complex cipher device, and it took Allied cryptographers many years to break it. However, once they did, it was a major turning point in the war. The Allies were able to read German intelligence messages, which gave them a significant advantage.
The Enigma machine is a fascinating example of the power of cryptography. It is a reminder that even the most secure ciphers can be broken, given enough time and resources.
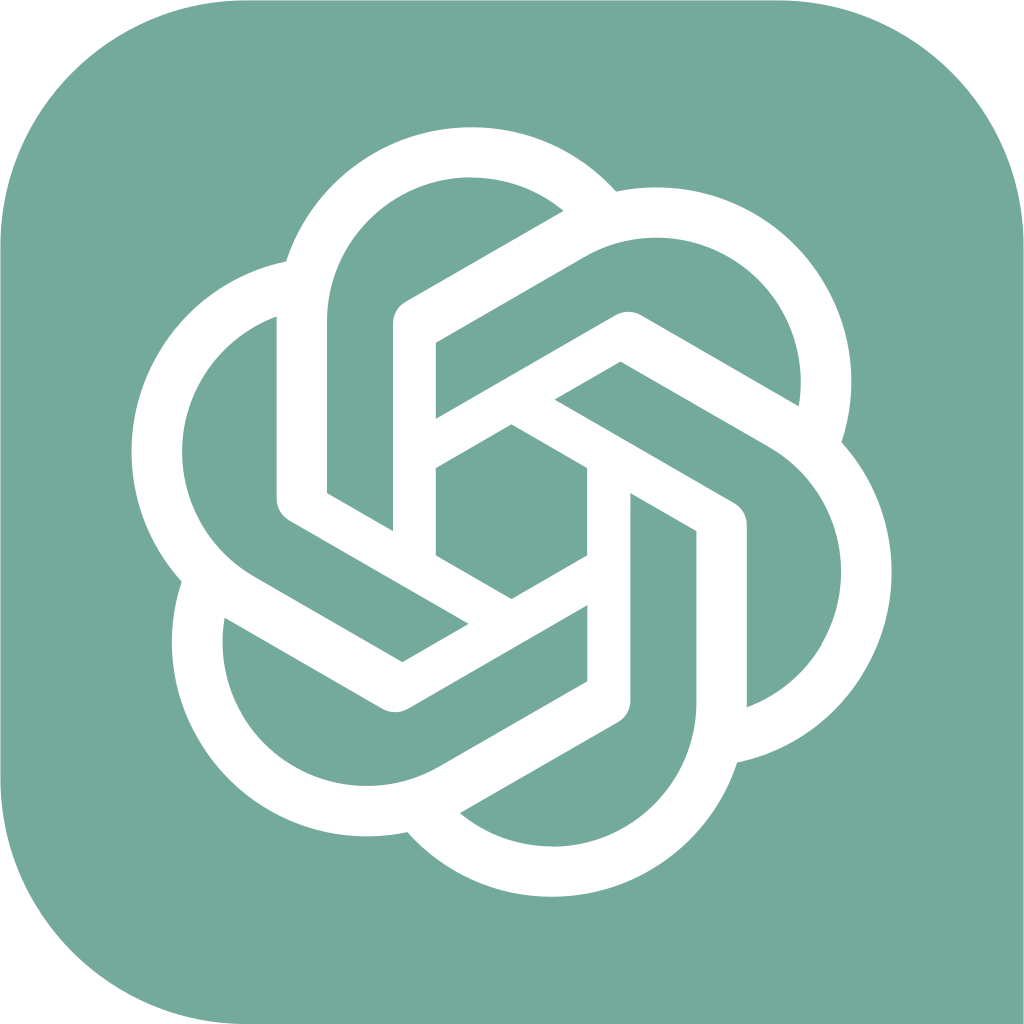
The text you provided appears to be encoded using the NATO phonetic alphabet. Decoding it, we get: Tango Hotel India Sierra = THIS India Sierra = IS November Alfa Tango Oscar = NATO Sierra Papa Echo Lima Lima India November Golf = SPELLING So, the decoded message is: "THIS IS NATO SPELLING"